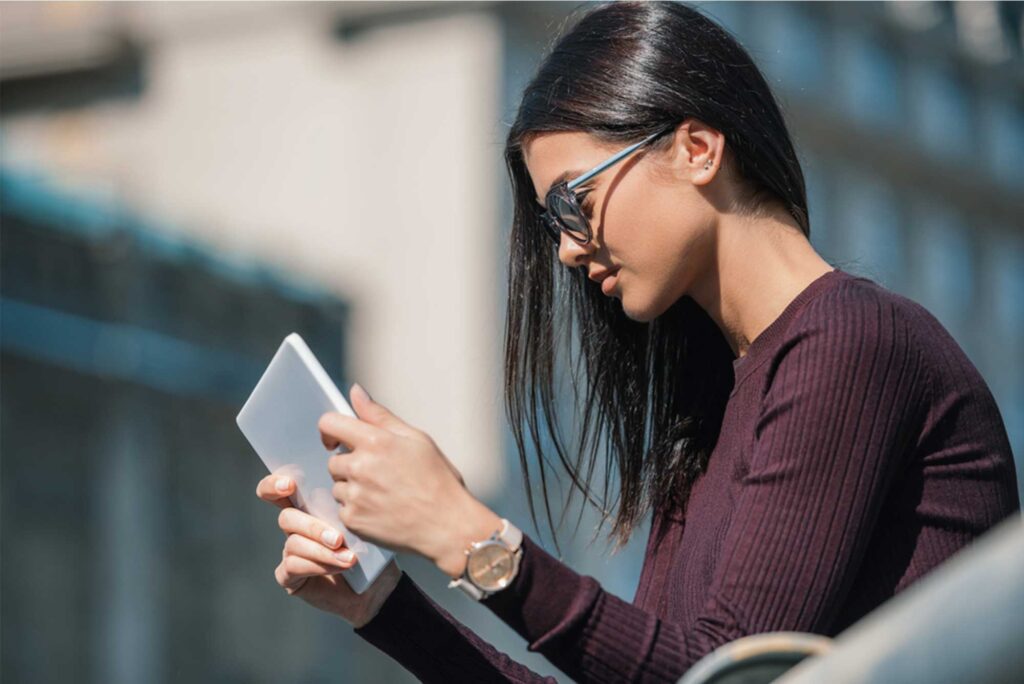
AP Computer Science Principles Exam Preparation
In this series of articles we will explain some of the sample questions that are provided to help AP CSP students help with exam preparation.
Programming
This article focuses on the AP CSP exam questions related to programming. This includes both coding questions that are written in pseudo code, and other questions related to programming such as good writing code practices, Boolean logic and programming languages.
Sample Exam Questions
We have taken examples from the sample questions from the two practice question sets from 2021 and from previous practice exercises provided by the college board.
Although it does not mean these are going to be in the exam, it is assumed that the primary areas of computing, programming, networks, data and algorithms will form most of the CSP exam.
These articles will present and explain questions and answers in these areas.
What type of programming questions will be in the CSP exam?
We don’t know. But we do know that during the AP CSP course we have learn about conditional if statements, nested if statements, loops, lists, list indexes and procedures.
In addition to the normal coding questions there are also Boolean logic with True and False, Not True, etc., Boolean expressions, some general questions about programming such as low or high level languages, and also robot movement questions – see below!
Practice Exam questions
Read the questions and check your answer with the explanation below. We want to help everybody so if you think the question is easy then move on to the next question without reading the solution if you wish.
Some questions have more than one answer – these multiple answer questions are also in the AP CSP exam.
Code
Each of the following can make an algorithm more readable except?
- A. Well-named variables and procedures
- B. Consistent formatting within the code
- C. Procedures that have one purpose
- D. Minimizing the use of loops so the program flow will be clearer
Which of these options is not a way of making the code more readable? More readable is true for A definitely, B also. C is no always true but it does help make the code easier to understand.
Option D might be an optimization concern but not readability. D is our answer.
Answer: D
Boolean expression
Which of these is NOT a Boolean expression?
- A. IF (eyes = brown AND height > 60)
- B. REPEAT UNTIL (song = favSong)
- C. IF (NOT ( pet = cat ) )
- D. x <- (x + 42)
Which of these options are True or False expressions and which one is not?
A is either T or F, B is not as straightforward but the brackets is a condition, C again is a condition, true or false. D is the odd one out, it is an assignment which adds 42 to x.
Answer: D
Boolean logic
Which of the following will evaluate to false?
i. false AND (true OR NOT (false) )
ii. true AND (NOT (true AND false ))
iii. NOT( false OR (true OR false) )
- A. i and ii
- B. ii and iii
- C. i and iii
- D. i, ii and iii
This is a lot easier if you know your tables for AND and OR. As you can see below 2 True = True and 2 False = False, but one of each is different for AND and OR.
AND
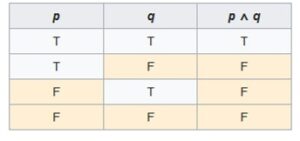
OR
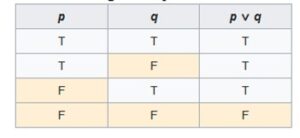
We think – if either is True then its True with OR, but if they are not both True then they are False with AND. So (true and false) in ii is false, but in iii (true or false) is true.
Lets look at each of the statements. We use T for True and F for False.
F +(T or T) means F + T, which is False
T + (NOT (F) ) is the same as T + T, which evaluates as True
F OR (T OR F) -> F OR T -> T, so NOT(T) is False
So we get i and iii as False
Answer: C
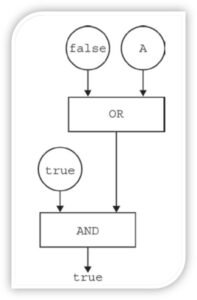
Which of the following is a true statement about input A?
- A. Input A must be true
- B. Input A must be false
- C. Input A can be either true or false
- D. There is no possible value of input A that will cause the circuit to have the output true
Answer: A
If-else statements (conditionals) and lists
IF topSongs[i] = song AND song_Times_Played = 100
REMOVE (topsongs, i)
ELSE
play (song)
song_Times_Played <- song_Times_Played + 1
Assume the variables and list already have values
What is the code doing?
- A. Plating a song from the topSongs list
- B. If a song is on topSongs, playing it, then removing it
- C. Removing song from topSongs if it has been played 100 times, otherwise playing the song and increasing the number of times played
- D. The code will not run as written
The code in English means if the song has played 100 times then remove it from the list, or, play the song and add one to the count of plays.
This is not straightforward. A is not wrong but the code does more than just play a song. It does not play then remove a song as in B.
C is the obvious answer. It does remove a song from topSongs when the counter reaches 100, and it plays the song and increases the variable as said.
But, wait, is there an error? This will delete a song after 100 plays of all songs. Confused?
Imagine if there are two songs and the first one plays 99 times. Then when song_Times_Played = 100 song two is the song. Then song two will be removed from the list although it has only been played once.
So C seems ok, but to keep a list of ‘times played’ for each song would require a list not a variable.
Answer: D
Nested if statements
IF onTime
DISPLAY ("Hello.")
ELSE
IF absent
DISPLAY ("Is anyone there?")
ESLE
DISPLAY ("Better late than never.")
If the variables onTime and absent both have the value false, what is displayed as a result of running the code segment?
- A. Is anyone there?
- B. Better late than never.
- C. Hello. Is anyone there?
- D. Hello. Better late than never.
Answer: B
Loops
i <- 0
sum <- 0
REPEAT UNTIL i = 4
i <- 1
sum <- sum + 1
i <- i + 1
DISPLAY (sum)
Which of the following best describes the result of running the program code?
- A. The number 0 is displayed
- B. The number 6 is displayed
- C. The number 10 is displayed
- D. Nothing is displayed; the program results in an infinite loop
Inside the loop, i will equal 1, then sum will equal 1, then i will equal 2. This will happen forever as i will never equal 4. An infinite loop.
Answer: D
Lists
#Block 1
REPEAT UNTIL x <= 0
{
APPEND (roster, name)
x <- x + 1
}
#Block 2
FOR EACH name IN roster
{
APPEND (roster, name)
DISPLAY (name)
}
Which code segment will correctly add a name to a team roster (assume all variables and lists are appropriately initialized)?
- A. Block 1
- B. Block 2
- C. Both Block 1 and Block 2
- D. Neither Block 1 and Block 2
Block 1 is wrong as x should decrease by 1 not increase. If x is over 0 then this will run forever, below and it will never run.
Block 2 duplicates the names already in a list. This does not ‘add’ a name to the roster. So both are incorrect.
Answer: D
FOR EACH score IN testScores
{
IF score > 0
{
total <- total + score
}
count <- count + 1
}
DISPLAY ("The average score on the test was:", total / count)
Test 1 [100, 90, 80, 80, 85, 50, 0, 85, 90]
Test 1 [192, 85, 74, 100, 96, 88]
If a programmer tested the above code with the values indicated, would the program correctly calculate the average of the test scores (assume all variables and lists are appropriately initialized)?
- A. Yes, the code works as it should for both test cases
- B. No, the code does not average the test scores correctly for either test
- C. No, the code only works for the Test 1 scores
- D. No, the code only works for the Test 2 scores
This code is good if the score is over 0. Test 1 includes 0 so it will omit that score and give a false average. Test 2 has valid numbers and therefore will be correct.
Answer: D
Procedures
PROCEDURE carMaint (miles)
{
checkUp <- false
IF (miles >= 4999)
{
checkUp <- true
}
RETURN (checkUp)
}
checkUp <- carMaint (4999)
What is the value of carChk after the code above runs?
- A. False
- B. True
- C. check up
- D. 4999
The procedure is passed 4999, the if statement is true so checkUp is true. So after the code is run the true value is returned to the variable carChk from the procedure.
Answer: B
More AP CSP exam practice questions
This is the third of a series of articles that take questions from the sample practice exams.
The first article was about computing questions. Click on the following link to see this article: Computing Questions in the Computer Science Principles AP CSP Exam
The second article was about network questions. Click on the following link to see this article: Network Questions in the Computer Science Principles AP CSP Exam
If you wish to see an overview of the sample questions related to the AP CSP exam, please visit the following article:
Ultimate Guide to AP Computer Science Principles Exam Questions
AP CSP exam webpage
For all courses, articles and information relating to the AP Computer Science Principles Exam, see our dedicated web page. Click the following link to visit this webpage: AP CSP Exam webpage
Big Ideas exam practice questions
There are also practice questions grouped by the ‘big ideas’ that are here in pdf format.
So, if you want free download AP CSP questions and answers, then click on the big idea: